Introduction to SPARQL
Once you have a dataset ready, you can visualize it in many ways – such as tables, tables with images, graphs, maps and many more.SPARQL (pronounced “Sparkle”) is a query language for databases stored in RDF format, such as the “triples” you see on the Linked Open Data page. SPARQL stands for “SPARQL Protocol and RDF Query Language”. SPARQL provides us the language to build data visualizations based on the results of queries run against the datasets.
The Wikidata SPARQL Enpoint
Wikidata offers an “endpoint” at https://query.wikidata.org, which is a graphical user interface that allows us to query and visualize the data in real-time. The SPARQL Endpoint facilitates writing out the code for each query.
One approach is to start by working with queries that run and modify them to learn how to use the interface. Queries that use data in the JJKB are found here.
If you hover over the left side of a data visualization with your mouse, you will see a menu of all of the data visualization types that are available for your specific data set. From here you can view your data in many different ways.
If you hover over the right side of the screen with your mouse, you will see a different set of options:
Edit Visually will allow you to work with the SPARQL code in a user interface:
… while Edit SPARQL opens up a window with the Wikidata Query Service containing the SPARQL code for the underlying query for your data visualization:
Help will lead you to the SPARQL help pages; Link provides a URL for your data visualization; and Download offers you several data formats to retrieve the data presented.
You select Examples or click on the Examples link
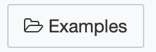
and select a query such as a list of paintings by Rembrandt in the Louvre or the Rijksmuseum:
Another way to work with the queries that the JJKB provides is to copy and paste the queries provided on the data visualization pages directly into the Wikidata Query Service; the SPARQL code for each query can be found at the bottom of its respective JJKB page, such as the following: http://artistarchives.hosting.nyu.edu/SPARQL_queries/JJKB_Mirage_OH_collaborators_IDs.htm#query.
Writing your own SPARQL queries
Start writing your queries following these steps:
1. Set the default view
What kind of data output would you like to see? To determine which output type will be shown when your query is run, use the code:
#defaultView:...
For example, you could start with a table:
#defaultView:Table
The Table view allows you to see all of the data which can be helpful in debugging either your query or evaluating inconsistencies that you are concerned about in the data. From here, you can experiment with other output formats to select one or more that best suit your particular data selection based on the query.
Note that if you wish to specify the default view, that must be in the first line.
Any other line that begins with a # will be ignored as a “comment” and the contents of the line will be displayed in a light gray as a reminder that those line(s) will not be executed as part of the query.
2. The SELECT clause
Next, which items would you like to see? Use SELECT for the data entities to display; in a table view, these are the columns that you wish to include. Here we are asking to see the item number, the item label and “instance of” (medium) label:
SELECT ?item ?itemLabel ?instance_ofLabel
3. The WHERE clause
This clause allows you to set the criteria to determine which items or entities in Wikidata you wish to include by setting up criteria. In a table view, these are the rows that you wish to include.
Note that for fixed values, wdt: signals a Wikidata property and wd: signals a Wikidata item:
?subject ?predicate ?object
For example:
?artwork wdt:P170 wd:Q453808.
To “translate” this into natural language, note that P170 is the property for creator as all properties are identified with a “P” and Q453808 is the “Q-number” for Joan Jonas as all identifiers begin with a “Q”. The clause
?artwork wdt:P170 wd:Q453808
“translates” into the following statement in English:
[Find] artworks [SUBJECT] whose creator [PREDICATE] is Joan Jonas [OBJECT].
Here is another example:
?artwork wdt:P1343 wd:Q91439317.
[Find] artworks [SUBJECT] described by [PREDICATE] the JJKB [OBJECT].
In order to see a “label” rather than simply a Q-number, include the following code in your WHERE clause:
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
For example, notice that if you run the following query without the SERVICE line, that the names of the exhibition locations (museums and galleries) remain blank!
#defaultView:Table
SELECT ?artwork ?artworkLabel ?inception ?start_time ?location ?locationLabel ?image
WHERE {
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
?artwork wdt:P170 wd:Q453808.
?artwork wdt:P1343 wd:Q91439317.
OPTIONAL { ?artwork wdt:P571 ?inception. }
OPTIONAL { ?artwork wdt:P580 ?start_time. }
?artwork wdt:P276 ?location.
OPTIONAL { ?location wdt:P18 ?image.}
}
To summarize the syntax:
- Each clause beginning with a SUBJECT is terminated by a period.
- Multiple PREDICATES for the same SUBJECT are separated by semicolons.
- Multiple OBJECTs for the same PREDICATE are separated by commas.
Sometimes a special node called a blank node is used. For example, here is a SPARQL query that lists an artwork, its description, and a floorplan reference. Note that prov:wasDerivedFrom is the predicate that points to a reference. In this case, we are using pr:854 which is the property reference that signals the use of a URL.
SELECT ?artwork ?artworkLabel ?artworkDescription ?floorplan_reference WHERE { SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". } ?artwork wdt:P1343 wd:Q91439317; wdt:P31 wd:Q838948; wdt:P527 wd:Q18965; p:P527 _:b21. _:b21 prov:wasDerivedFrom _:b20. _:b20 pr:P854 ?floorplan_reference.
Here is how you could “translate” this into English:
Show us the artwork ID, name, description and a link to its floor plan
In all cases WHERE
The artwork field ([subject] is described by [predicate] the JJKB [object]
AND this artwork is an instance of a work of art
AND this artwork has a part which is a floorplan
AND that part has a reference [which is a URL].
Use MINUS to exclude entities. For example, this query omits artworks that are not associated with a location. For those that have a location, the corresponding image is also displayed.
#defaultView:Timeline
SELECT ?item ?itemLabel ?instance_ofLabel ?location ?locationLabel ?image ?inception ?start_time WHERE {
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
?item wdt:P1343 wd:Q91439317;
wdt:P31 ?instance_of.
MINUS { ?item wdt:P31 wd:Q838948. }
?item wdt:P276 ?location.
OPTIONAL { ?location wdt:P18 ?image. }
OPTIONAL { ?item wdt:P571 ?inception. }
OPTIONAL { ?item wdt:P580 ?start_time. }
}
4. LIMIT
Use LIMIT if you are concerned about how much data will be returned. For example, if you wish to only display the first 25 results found in a query, this case a table of all of the artworks for Organic Honey and for Mirage described by the Joan Jonas Knowledge Base on Wikidata:
#defaultView:Table
SELECT ?artwork ?artworkLabel ?performer ?performerLabel ?contributor_to_the_creative_work_or_subject
?contributor_to_the_creative_work_or_subjectLabel ?object_has_role ?object_has_roleLabel
WHERE {
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
?artwork wdt:P1343 wd:Q91439317;
wdt:P31 wd:Q838948.
}
LIMIT 25
5. ORDER BY
Use ORDER BY to sort the results and specify ASC( … ) or DESC( … ) for ascending or descending, for example to see the above table in order by the artwork title (label):
#defaultView:Table
SELECT ?artwork ?artworkLabel ?inception ?start_time ?location ?locationLabel ?image
WHERE {
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
?artwork wdt:P170 wd:Q453808.
?artwork wdt:P1343 wd:Q91439317.
OPTIONAL { ?artwork wdt:P571 ?inception. }
OPTIONAL { ?artwork wdt:P580 ?start_time. }
?artwork wdt:P276 ?location.
OPTIONAL { ?location wdt:P18 ?image.}
}
ORDER BY ASC(?artworkLabel)
Note that OPTIONAL means that you will see results even if not all of the associated information is available. Without OPTIONAL, the query above does not return any results because every artwork is missing at least one of the associated values. It is a good idea to use OPTIONAL with separate clauses so that you will see results with any combination of partial data!
6. GROUP BY and COUNT
Use COUNT( ) and GROUP BY to build aggregate queries. For example, to find out how many Organic Honey and Mirage artworks are located at each of the locations specified in our dataset, use the following query:
#defaultView:Table
SELECT ?location ?locationLabel (COUNT(*) AS ?count) ?image
WHERE {
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
?artwork wdt:P170 wd:Q453808.
?artwork wdt:P1343 wd:Q91439317.
?artwork wdt:P276 ?location.
OPTIONAL { ?location wdt:P18 ?image.}
}
GROUP BY ?location ?locationLabel ?image
ORDER BY DESC(?count) ASC(?locationLabel)
Tools
Use these tools to help you write your queries:

The “query helper” offers an additional window with suggestions.
Opens the query window to the full screen.
The prefix defaults to Wikidata: ‘wd’ for Wikidata entity and ‘wdt’ for Wikidata property .
Formats the query for readability (a “beautify” tool).
Opens the examples folder.
Retrieves a previous query.
Clears the query.
Builds a URL to this query.
Run your query.
Resources
Tutorial
Video Tutorial on SPARQL for studying the work of Joan Jonas
Further Reading
- DuCharme, Bob. Learning SPARQL: Querying and Updating with SPARQL 1.1. Second edition. Sebastopol, CA: O’Reilly Media, 2013.
- Feigenbaum, Lee. SPARQL by Example: A Tutorial by Lee Feigenbaum.
- Feigenbaum, Lee. SPARQL BY EXAMPLE: The Cheat Sheet.
- Graves, Alvaro. “Tech: A Crash Course in SPARQL”.
- Heath, Sebastian. “SPARQL as a First Step for Querying and Transforming Numismatic Data: Examples from Nomisma.Org.” In Alexander the Great. A Linked Open World, edited by S. Glenn, A. Meadows, and F. Duryat, 35–52. Bordeaux, France: Ausonius Éditions, 2018.
Wikidata Documentation and Tutorials on SPARQL
- Request a Query https://www.wikidata.org/wiki/Wikidata:Request_a_query
- Wikidata Query Help https://www.wikidata.org/wiki/Wikidata:SPARQL_query_service/Wikidata_Query_Help
- Wikidata Query Service https://query.wikidata.org
- Wikidata SPARQL Introduction https://www.wikidata.org/wiki/Wikidata:SPARQL_query_service#Learning_SPARQL
- Wikidata SPARQL Tutorial https://www.wikidata.org/wiki/Wikidata:SPARQL_tutorial